Extending Burp Suite
Introduction
There are multiple intercepting proxy tools available and Burp Suite is one of the best tools available for interception. If you are not yet familiar with it, for a brief Burp Suite Walkthrough, please read the article written by Prateek Gianchandani.
The added advantage Burp provides is its extensible functionality with the IBurpExtender interface and others like IBurpExtenderCallbacks , IScanIssue, etc. Burp Extensions can read and manipulate Burp's runtime data and configuration and send it to various Burp tools such as Repeater, Scanner, and Intruder.
We will try and understand what each interface does and then in the later part of the article, we will see how various interfaces interact with the help of an example.
Burp Interfaces
IBurpExtender:
This interface forms the base of the BurpSuite extension. All the Burp extensions provide an implementation of this class by implementing at least one method of IBurpExtender interface.
It can be implemented by declaring a public class called "BurpExtender". When we start Burp, it looks for a class BurpExtender in its classpath to load and instantiate. This class is supposed to implement the IBurpExtender interface.
After BurpExtender is loaded during startup, whenever Burp Proxy receives a client request or server response it invokes the function "processProxyMessage" (if declared in the BurpExtender class).
IBurpExtenderCallbacks:
Another important interface to note while working on BurpSuite extension is the IBurpExtenderCallbacks interface. Its main purpose is to pass callback methods to the IBurpExtender interface implementation class (BurpExtender). It links the Burp tools like Repeater, Proxy, Intruder, etc., with the BurpExtender class with the help of functions like sendToRepeater, sendtoIntruder, etc.
The IBurpExtender interface has a method "registerExtenderCallbacks". If BurpExtender (an implementation of IBurpExtender) implements this method, it receives an implementation of the IBurpExtenderCallbacks interface. Hence, it can call any of the methods described in the IBurpExtenderCallbacks.
Using the callbacks interface, we can send requests to repeater/intruder, send alerts to the Alert tab, issue arbitrary requests and retrieve responses.
IHttpRequestResponse:
All the HTTP messages captured with the Burp or Burp extension have details associated with it like host, port, request, response etc. Its two set of methods, getter and setter methods; helps to get all the information or modify the message if needed.
The IBurpExtender interface has a method "processHTTPMessage" which is invoked each time any of the Burp tools sends a client request or receives a response. Here, we can get the details about the HTTP Message using the getter methods or by modifying the parameters of the request using the setter methods. This modified request can then be forwarded to any of the Burp tools i.e., Repeater, using callback methods.
IScanIssue:
The IScanIssue interface is used to get the details of the issue like Severity, Confidence, URL, Issue Detail etc. which are reported by the Burp Scanner.
The IBurpExtender interface provides a method 'newScanIssue' which is invoked each time Burp Scanner discovers/reports a new issue. So if your BurpExtender implementation has implemented this method, every time there is a new issue you can perform some action on it i.e., extra logging, report generation or automatic bug filing.
IScanQueueItem:
Burp supports two type of scanning, namely active and passive scanning. IScanQueueItem helps Burp extensions to access the "Active Scan" queue and retrieve more information like the number of issues reported, issue details, percentage completed till now, insertion points, etc.
IBurpExtenderCallbacks provide methods like 'doActiveScan' which can be used to send HTTP Request to the Burp Scanner to perform an active vulnerability scan. This method returns an IScanQueueItem object and using this interface, you can find out the details about the active scan queue.
IMenuItemHandler:
This interface provides user the capability to write custom context menus and define a handler for it. There are two steps here while implementing the custom menu, first being the extensions must provide an implementation of the registerMenuItem method of IBurpExtenderCallbacks to register each custom menu item. Also, there should be a class implementing the IMenuItemHandler interface and its method "menuItemClicked". As the name suggests, the method is called when the user clicks on the custom menu item.
In the example shown below, our motive is to provide the user a menu option to save the response which is currently being displayed on the screen.
Compiling and Running the Extension
People often face issues while compiling and running Burp Extension.
Let's consider the example of MenuItemHandler to see how we can run the extension.
Pre-requisite: You need to have JDK and Burp installed on your system.
- Import burp.*;
- D:burp_extension>"C:Program FilesJavajdk1.6.0_29binjavac.exe" BurpExtender.java
- D:burp_extension>"C:Program FilesJavajdk1.6.0_29binjar.exe" -cf burpextender.jar BurpExtender.class extendedMenuItem.class
- D:burp_extension>java -Xmx512m -classpath burpextender.jar;burpsuite_pro_v1.4.04.jar burp.StartBurp
To confirm that the BurpExtension was loaded successfully, check the Alerts tab of the BurpSuite. In the screenshot below you can read the message which says method "registerExtenderCallbacks" found.
The 'alerts' tab only shows that the BurpExtender class was successfully loaded, but let us check if a custom context menu created.
Go to 'proxy' and then the 'history' tab, and for any request captured right-click on the screen. A context menu will appear on the screen and you can notice, the context menu "Capture The Response" has been created by us.
Also, at the command line, when BurpSuite is started, we can see the statement we had printed in the constructor of the ExtendedMenuItem.
Now, when we click on "Capture the Response", we can see the response being captured on the output screen (command line).
Alternate Implementation:
There can be multiple uses of this extension, including increased logging functionality, generating reports, capturing some particular request/response, information gathering, high-level functions like integrating CAPTCHA forms, etc.
Consider another example. This time our motive is to process every server response in Burp Proxy and gather all the email IDs and comments in the response and save the output to a file.
We will implement the method processProxyMessage (Block 1 in the diagram below) and process only responses (Block 2 in the diagram below). We want to process only the responses which are in Suite scope; hence we will make use of the callback "isInScope" provided by the IBurpExtenderCallbacks interface. Once we extract the response body from the response, we can use Java regular expressions and patterns to grep for comments and email IDs in the body.
ProcessEmailComments is the method to find out the email IDs and comments in the response which then calls the SavetoFile method to save the output in two different files.
In the example I ran the output on the command line was:
Two files were created as shown below:
For the source code of the different interfaces mentioned in this article please visit:
11 courses, 8+ hours of training
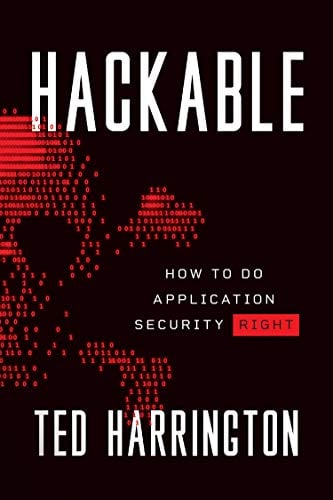
11 courses, 8+ hours of training
- http://portswigger.net/burp/extender/IBurpExtender.java
- http://portswigger.net/burp/extender/IBurpExtenderCallbacks.java
- http://portswigger.net/burp/extender/IHttpRequestResponse.java
- http://portswigger.net/burp/extender/IScanIssue.java
- http://portswigger.net/burp/extender/IScanQueueItem.java
- http://portswigger.net/burp/extender/IMenuItemHandler.java
For the source code in the examples mentioned above, please leave me a comment.