Input Validation Course
5 hours, 23 minutes
Course description
Lack of input validation is the single most commonly cited mistake that mobile app developers make. Corrupt or manipulated input lies at the root of most malicious hacking exploits. As a mobile app developer, you need to know how to defend your app and the user's data from attack. In this course you will learn which characters can be misinterpreted as commands, and how to render those characters harmless. You will practice using a number of input sanitization and techniques including regular expressions and Swift functions. You'll defend against SQL injection, understand the larger scope of cross-site scripting and cross-site request forgeries, and validate the identity of a website API whose content your app consumes. You will also learn how to defend against unexpected attack vectors such as QR codes and deserialized JSON objects.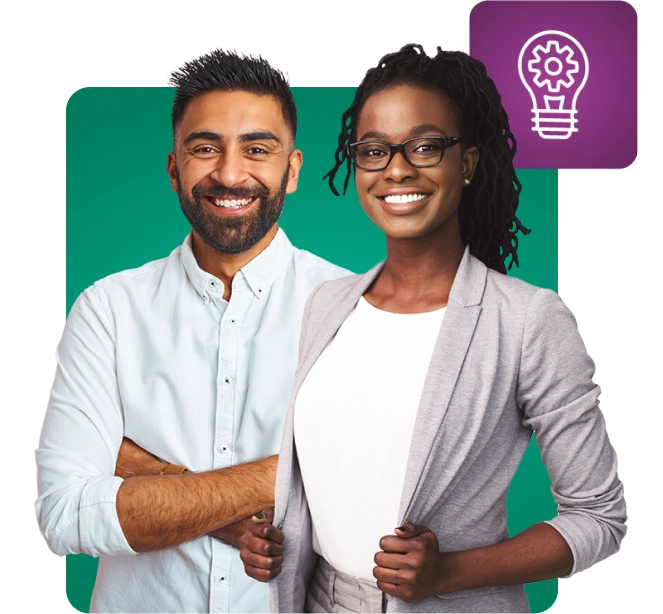
Syllabus
Activity: Protecting users against insecure UIWebView
Video - 00:11:00
WebView protection
Video - 00:05:00
Activity: Securely working with JSON, part 2
Video - 00:09:00
Activity: Securely working with JSON
Video - 00:13:00
Activity: Installing Alamofire and SwiftyJSON pods
Video - 00:04:00
Object deserialization
Video - 00:07:00
SQL injection, part 2
Video - 00:04:00
SQL injection
Video - 00:11:00
Activity: Filtering a malicious QR code, part 2
Video - 00:06:00
Activity: Filtering a malicious QR code
Video - 00:12:00
Code injection
Video - 00:15:00
Activity: Exploring XSS attacks
Video - 00:10:00
Cross-site attacks
Video - 00:12:00
Null bytes
Video - 00:07:00
Activity: Sanitizing input with a property wrapper
Video - 00:07:00
Activity: Value clamping with a property wrapper
Video - 00:07:00
Activity: Trimming whitespace and newlines with a property wrapper
Video - 00:07:00
Property wrappers
Video - 00:09:00
Activity: Sanitizing input, part 2
Video - 00:14:00
Activity: Sanitizing input
Video - 00:11:00
Activity: Regular expressions, part 3
Video - 00:08:00
Activity: Regular expressions, part 2
Video - 00:07:00
Activity: Regular expressions
Video - 00:08:00
Input sanitization - Regular expressions, part 2
Video - 00:07:00
Input sanitization - Regular expressions
Video - 00:08:00
Input sanitization
Video - 00:13:00
Activity: Playing with format strings
Video - 00:10:00
Format string attack, part 3
Video - 00:07:00
Format string attack, part 2
Video - 00:09:00
Format string attack
Video - 00:08:00
Special characters, Part 2
Video - 00:07:00
Special characters
Video - 00:14:00
Activity: Disabling AutoCorrection
Video - 00:11:00
AutoCorrect and AutoFill
Video - 00:11:00
Understanding input risks
Video - 00:14:00
Unlock 7 days of free training
- 1,400+ hands-on courses and labs
- Certification practice exams
- Skill assessments
Associated NICE Work Roles
All Infosec training maps directly to the NICE Workforce Framework for Cybersecurity to guide you from beginner to expert across 52 Work Roles.
- All-Source Analyst
- Mission Assessment Specialist
- Exploitation Analyst
Plans & pricing
Infosec Skills Personal
$299 / year
- 190+ role-guided learning paths (e.g., Ethical Hacking, Threat Hunting)
- 100s of hands-on labs in cloud-hosted cyber ranges
- Custom certification practice exams (e.g., CISSP, Security+)
- Skill assessments
- Infosec peer community support
Infosec Skills Teams
$799 per license / year
- Team administration and reporting
- Dedicated client success manager
-
Single sign-on (SSO)
Easily authenticate and manage your learners by connecting to any identity provider that supports the SAML 2.0 standard.
-
Integrations via API
Retrieve training performance and engagement metrics and integrate learner data into your existing LMS or HRS.
- 190+ role-guided learning paths and assessments (e.g., Incident Response)
- 100s of hands-on labs in cloud-hosted cyber ranges
- Create and assign custom learning paths
- Custom certification practice exams (e.g., CISSP, CISA)
- Optional upgrade: Guarantee team certification with live boot camps
Award-winning training you can trust



